The Real Cause of S3 SignatureDoesNotMatch (And Why You're Debugging the Wrong Thing)
If you work with Amazon S3, you've likely encountered the SignatureDoesNotMatch error at some point. AWS gives you a cryptic message about mismatched signatures, and you start diving into your application code, checking SDK calls, logging headers, and tweaking IAM permissions—only to end up frustrated after hours of debugging.
Here’s the thing: you’re probably debugging the wrong part of your stack.
Most developers assume the issue is in their application logic. But more often than not, the root cause is an issue with how the request is signed, not what your app is doing. And the best way to verify that? Test a minimal request in Postman or Insomnia first.
What Causes the S3 SignatureDoesNotMatch Error?
AWS authenticates every API request by comparing a calculated signature (based on your request details) with the signature you provide. If they don’t match, AWS rejects the request with a 403 Forbidden and the dreaded SignatureDoesNotMatch error.
This can happen due to:
- Clock skew: Your local machine’s time is out of sync.
- Incorrect credentials: A mismatched or incomplete access key/secret key.
- Wrong signing method: Using Signature V2 instead of Signature V4 (or vice versa).
- Extra or missing headers: Signed headers differ from the ones sent in the actual request.
- Incorrect region: Your request is being sent to the wrong S3 region.
- Broken URL encoding: Special characters in your request are encoded incorrectly.
The Fastest Way to Debug S3 SignatureDoesNotMatch
Before wasting hours on your application code, strip everything down to a minimal request using Postman or Insomnia.
Step 1: Generate a Presigned URL
Use the AWS CLI to generate a presigned URL for an S3 object.
aws s3 presign s3://your-bucket-name/test-object.txt --region us-east-1
This gives you a temporary, signed URL that should work immediately in Postman or Insomnia.
Step 2: Test the Presigned URL
Open Postman or Insomnia, paste the presigned URL, and send a GET request.
- If it works → Your credentials and AWS setup are fine. The issue is in your app.
- If it fails → You have a signing or configuration issue.
Step 3: Manually Sign a Request
If the presigned URL works but your app still fails, try manually signing a request.
Using AWS SDK for JavaScript (v3):
const { S3Client, GetObjectCommand } = require("@aws-sdk/client-s3"); const client = new S3Client({ region: "us-east-1", credentials: { accessKeyId: "YOUR_ACCESS_KEY", secretAccessKey: "YOUR_SECRET_KEY", }, }); async function fetchObject() { try { const command = new GetObjectCommand({ Bucket: "your-bucket", Key: "test-object.txt" }); const response = await client.send(command); console.log("Success!", response); } catch (error) { console.error("Error:", error); } } fetchObject();
Step 4: Verify Your SDK Configuration
Some AWS SDKs default to older signing methods (Signature V2 instead of V4). Make sure you're explicitly setting signatureVersion: 'v4'
where necessary.
For Node.js AWS SDK (v2):
const s3 = new AWS.S3({ signatureVersion: 'v4' });
For Python (Boto3):
s3 = boto3.client('s3', config=Config(signature_version='s3v4'))
Step 5: Check for Common Configuration Issues
- Check Your System Clock
- Run
date
(Linux/macOS) orw32tm /query /status
(Windows). - If it's off by more than a few seconds, sync it with an NTP server.
- Run
- Ensure You’re Using the Correct Region
- Run
aws s3api get-bucket-location --bucket your-bucket-name
- If the output says
"LocationConstraint": "us-west-2"
, but your requests go tous-east-1
, that’s your problem.
- Run
- Remove Hardcoded Credentials (If Possible)
- AWS SDKs can automatically pick up credentials from environment variables:
export AWS_ACCESS_KEY_ID="your-access-key" export AWS_SECRET_ACCESS_KEY="your-secret-key"
- Or use the AWS shared credentials file (
~/.aws/credentials
).
- AWS SDKs can automatically pick up credentials from environment variables:
Conclusion: Debug Smarter, Not Harder
Most S3 SignatureDoesNotMatch errors are due to subtle misconfigurations, not actual bugs in your application. Before tearing apart your code, try the following:
- Generate a presigned URL and test it in Postman or Insomnia.
- Manually sign a request in your programming language’s AWS SDK.
- Verify your region, credentials, and system time.
By focusing on AWS’s signing process before diving into your code, you can fix these errors in minutes instead of hours. Debug smarter, not harder!
Final Tip
If you’re still stuck, check the AWS CloudTrail logs to see what AWS actually received versus what you thought you sent. Sometimes, the difference is just a single missing header.
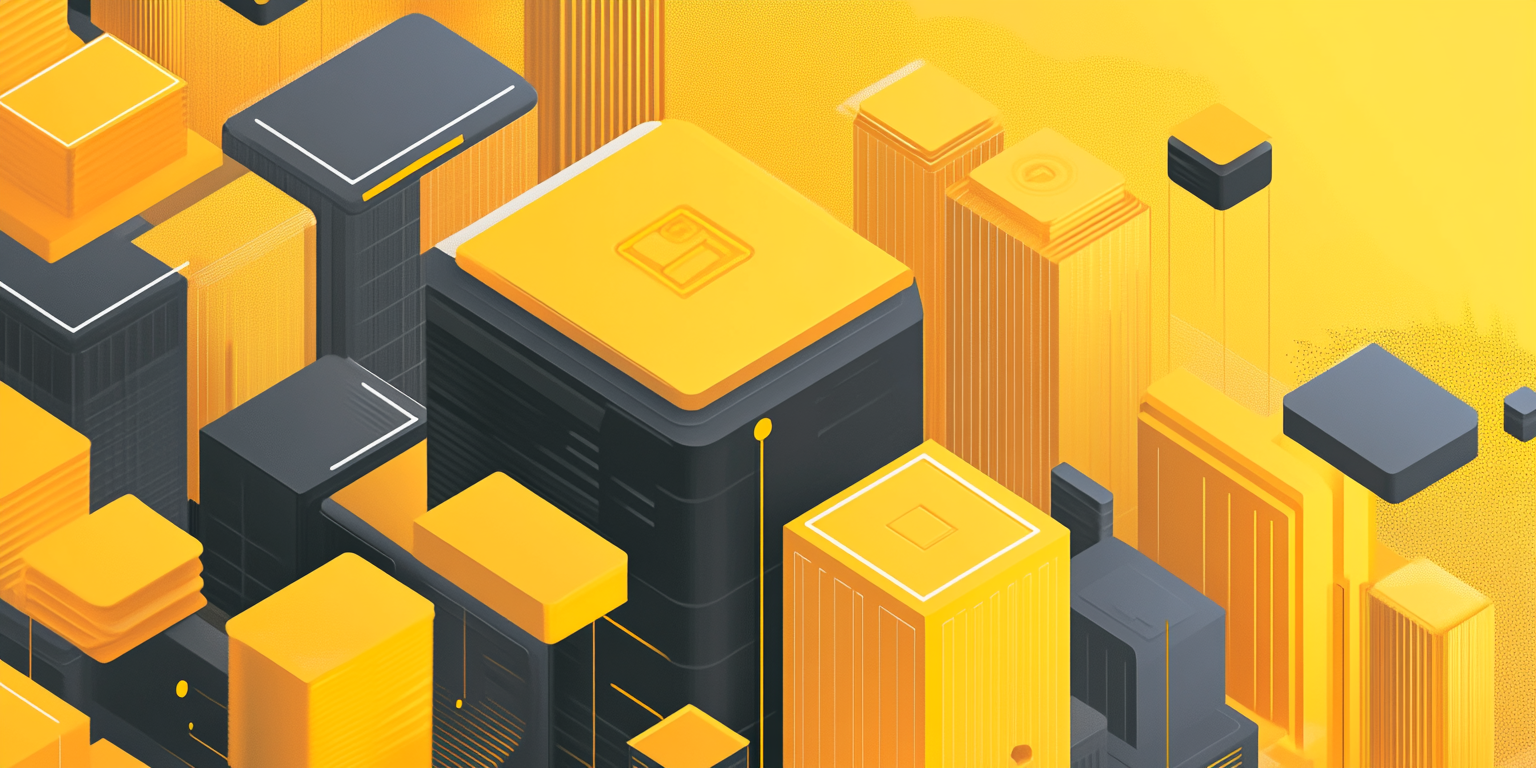
S3 SignatureDoesNotMatch errors can be daunting