Abstracting Away from Object Storage Like S3 is Always a Good Idea
Working directly with object storage like Amazon S3 might seem convenient at first, but it quickly becomes a bottleneck for local development, testing, and portability. A better approach is to introduce a storage abstraction layer that allows you to work with different storage backends—whether it's a local filesystem for development, S3 in production, or even another cloud provider.
With an abstraction in place, developers can test using mocks, run the same code across environments, and avoid vendor lock-in. This flexibility is crucial for modern software development, where seamless transitions between local, staging, and production environments are key to an efficient CI/CD pipeline.
A Unified Interface Across Environments
A well-designed storage abstraction ensures that your application can handle local files during development while using S3 or other cloud storage providers in production. Instead of maintaining separate logic for different storage backends, an abstraction layer lets you swap implementations effortlessly.
For instance, a Python-based app can work with local disk storage in development and seamlessly switch to S3, Google Cloud Storage, or Azure Blob Storage in production, all without modifying core application logic.
Easier and More Reliable Testing
One of the biggest challenges with object storage is testing. Making real API calls to S3 during unit tests is slow, costly, and unreliable. By introducing storage abstraction, you can easily mock file operations, enabling fast and isolated tests.
For example, in Go, the afero
library provides an in-memory filesystem that can be used for unit tests, eliminating dependencies on real storage services. Similarly, Python’s Cloudstorage
and JavaScript’s abstract-fs
provide ways to handle file storage through a mockable interface.
Libraries for Object Storage Abstraction
Instead of writing custom wrappers, you can use well-established libraries that provide object storage abstraction across different languages:
- PHP: Flysystem - A flexible abstraction layer for multiple storage backends, including local filesystems, AWS S3, and more.
- Python: Apache Libcloud - A unified API for interacting with various cloud storage providers.
- JavaScript/Node.js: abstract-fs - A modular filesystem abstraction layer.
- Ruby: CarrierWave - A flexible way to handle file uploads with support for cloud storage backends.
- Java: Apache Commons VFS - Provides a single API for accessing local and remote filesystems.
- Go: afero - A powerful abstraction for handling filesystem operations in Go.
- Rust: vfs - A virtual filesystem abstraction for Rust.
By integrating these libraries into your project, you can avoid reinventing the wheel while maintaining a clean and maintainable storage layer.
Implementing Object Storage Abstraction
Here’s an example in Python using Apache Libcloud
to create a storage abstraction:
from libcloud.storage.types import Provider from libcloud.storage.providers import get_driver class StorageBackend: def upload(self, filename, data): raise NotImplementedError def download(self, filename): raise NotImplementedError class LocalStorage(StorageBackend): def upload(self, filename, data): with open(f"/tmp/{filename}", "wb") as f: f.write(data) def download(self, filename): with open(f"/tmp/{filename}", "rb") as f: return f.read() class S3Storage(StorageBackend): def __init__(self, key, secret, bucket_name): cls = get_driver(Provider.S3) self.driver = cls(key, secret) self.bucket = self.driver.get_container(container_name=bucket_name) def upload(self, filename, data): obj = self.driver.upload_object_via_stream(iterator=[data], container=self.bucket, object_name=filename) return obj def download(self, filename): obj = self.driver.get_object(container_name=self.bucket.name, object_name=filename) return obj.download() # Switching between local and S3 dynamically import os if os.getenv("USE_S3"): storage = S3Storage("your-key", "your-secret", "your-bucket") else: storage = LocalStorage()
With this setup, your application can switch between local storage and S3 just by changing an environment variable—without modifying any application logic.
No Vendor Lock-In
Hardcoding your storage operations to a single provider means migrating in the future could be painful. Abstracting object storage ensures that you can move from S3 to Google Cloud Storage, Azure Blob Storage, or even an on-premise solution like MinIO without rewriting your application.
Conclusion
By abstracting object storage, you make your application more portable, testable, and resilient. Whether you’re running locally, in staging, or in production, a unified storage interface ensures consistent behavior across all environments.
With libraries like Flysystem, Libcloud, Afero, and CarrierWave, implementing this abstraction is straightforward, allowing your team to focus on building features rather than dealing with storage complexities.
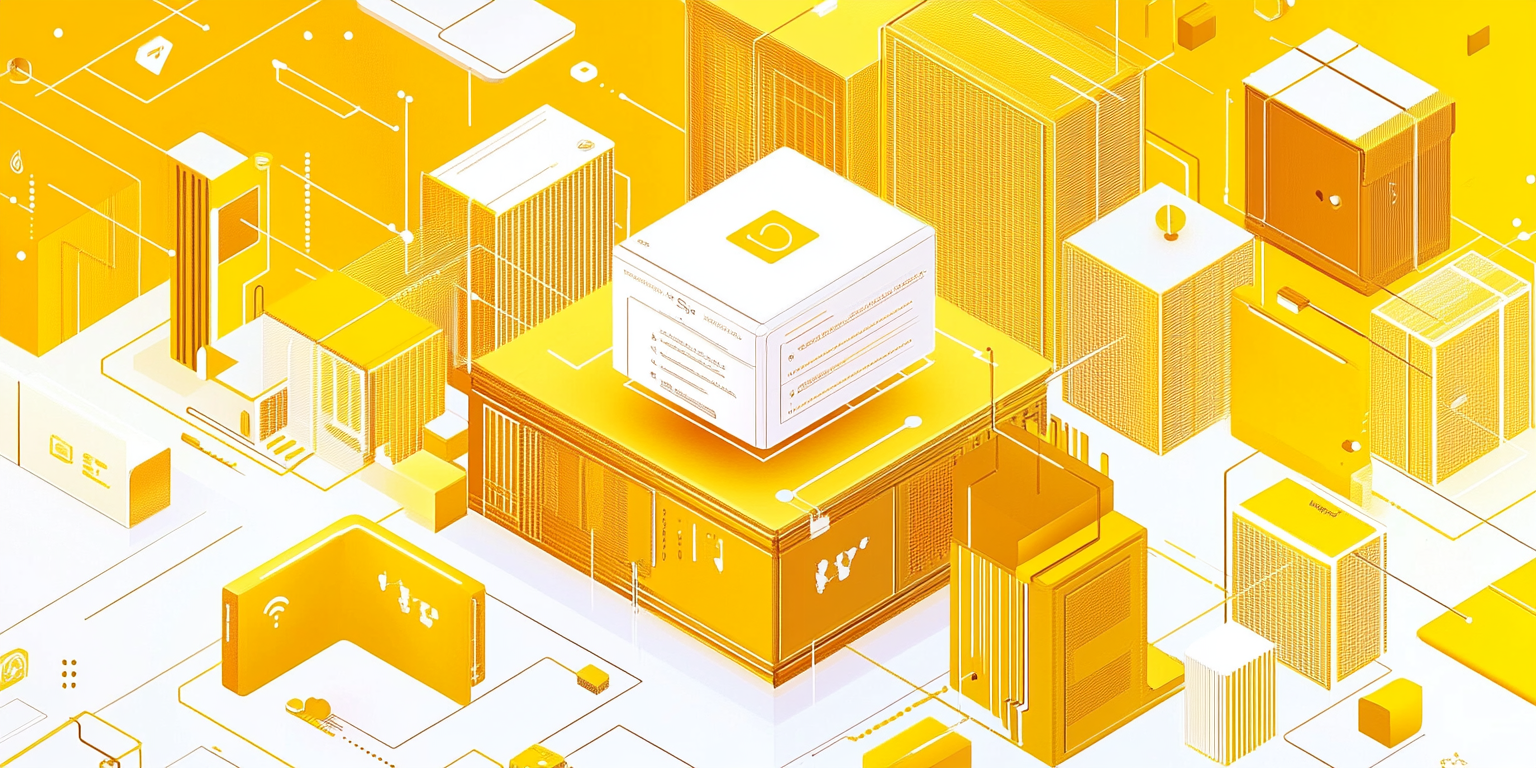
Storage abstractions make your application easier to maintain